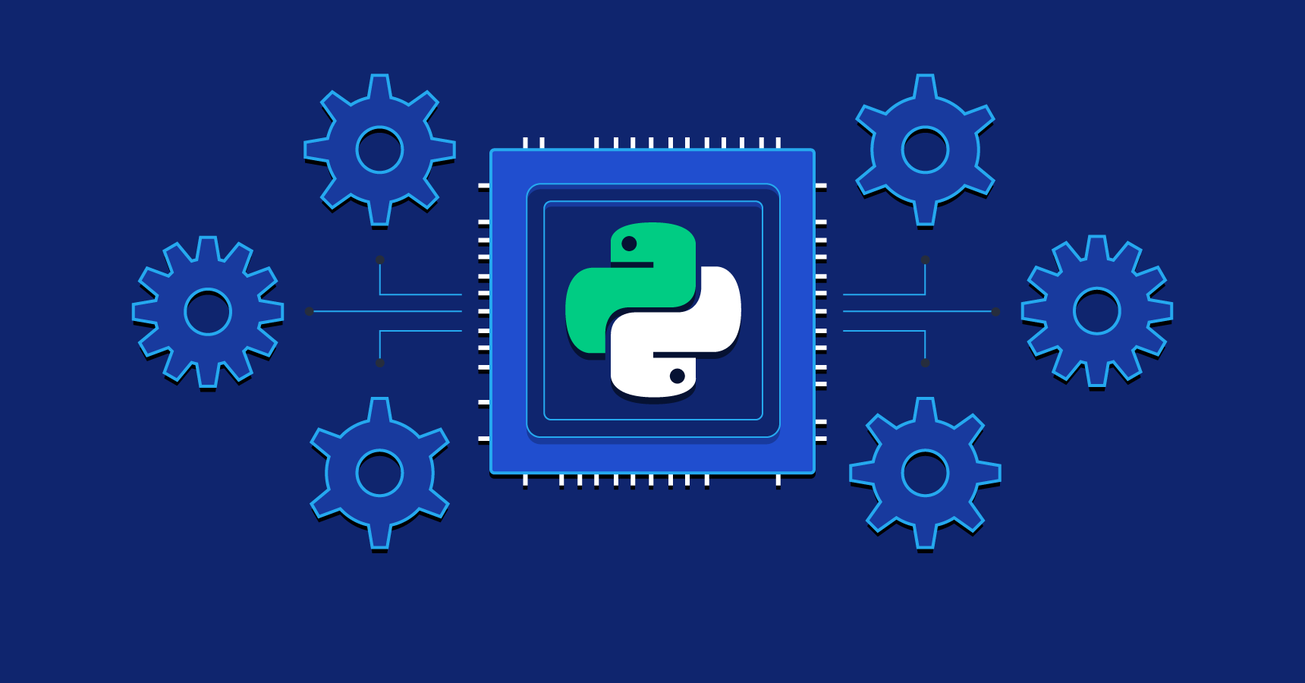
Multithreading is a programming technique where multiple threads are executed concurrently within a single program or process. Threads are essentially smaller units of a process that can run independently but share the same resources, like memory. This allows for more efficient utilization of CPU resources, especially in applications where multiple tasks can run simultaneously.
Key Concepts in Multithreading:
- Thread:
- A thread is a smaller sequence of programmed instructions that can be managed independently by a scheduler. In a multithreaded program, multiple threads share the same memory space and resources.
- Concurrency vs. Parallelism:
- Concurrency refers to the ability of a program to deal with multiple tasks at once, potentially by switching between them. Parallelism, on the other hand, involves executing multiple threads simultaneously, typically on multiple CPU cores. Multithreading helps achieve both, depending on the hardware and OS capabilities.
- Use Cases:
- Improving Performance: Multithreading is often used in applications that can be broken into smaller, independent tasks, like handling web server requests, real-time games, or GUI applications that need to stay responsive.
- I/O Bound Programs: Programs that spend a lot of time waiting for input/output operations (e.g., reading files or making network requests) benefit significantly from multithreading since one thread can continue while another waits.
Benefits of Multithreading:
- Increased Efficiency:
- By using multiple threads, programs can perform tasks more efficiently, taking advantage of modern multi-core processors.
- Responsiveness:
- Multithreading is useful for applications like GUIs, where one thread can handle user interactions, while others handle tasks in the background, keeping the application responsive.
- Resource Sharing:
- Threads within the same process share the same memory space, which makes communication between threads easier and reduces the overhead of managing separate memory areas.
Challenges and Considerations:
- Synchronization:
- Since threads share memory, it’s possible for multiple threads to access and modify the same data simultaneously, which can lead to data inconsistency. Synchronization mechanisms like locks, semaphores, and monitors are used to control access to shared resources and prevent issues like race conditions.
- Thread Safety:
- A program or piece of code is considered thread-safe if it functions correctly during simultaneous execution by multiple threads. Ensuring thread safety requires careful coding and the use of synchronization techniques.
- Deadlock:
- Deadlock occurs when two or more threads are waiting for resources held by each other, causing all of them to stall indefinitely. Proper resource management and avoiding circular dependencies help in preventing deadlocks.
- Context Switching:
- When the CPU switches between threads, it incurs a performance cost known as context switching. Although multithreading can improve performance, excessive context switching can lead to inefficiencies.
- Complexity:
- Writing multithreaded programs is more complex than writing single-threaded ones. Debugging can also be difficult due to unpredictable thread scheduling and synchronization issues.
Tools and Languages:
- Many modern programming languages provide built-in support for multithreading, including Java, Python, C++, and C#. Each language provides its own set of libraries or frameworks to create and manage threads.
- Java: Has the
Thread
class andRunnable
interface, along with newer features like the ExecutorService for managing thread pools. - Python: Uses the
threading
module for multithreading, but because of the Global Interpreter Lock (GIL), true parallel execution is often limited (useful for I/O-bound tasks). - C++: Provides the
<thread>
library in C++11 for multithreading, making it easier to create and manage threads compared to older approaches.
- Java: Has the
When to Use Multithreading:
- I/O-Bound Programs: Multithreading is highly beneficial for applications that involve a lot of waiting, like reading files, network requests, or interacting with databases.
- Maintaining Responsiveness: In GUI applications, where one thread can handle user input while another performs background operations.
- Real-Time Processing: For tasks that need to be processed quickly without waiting for the previous task to complete.
When to Avoid Multithreading:
- CPU-Bound Tasks with Limited Cores: If the program involves intensive calculations and there are fewer CPU cores available, adding more threads may lead to excessive context switching without real performance gains.
- Complex Synchronization: If managing shared resources between threads is too complex, it could lead to more bugs and require extensive debugging.
Multithreading is a powerful way to improve the performance and responsiveness of applications, especially with multi-core CPUs. However, it’s crucial to manage complexity, synchronization, and potential pitfalls like deadlocks and race conditions to ensure a stable, efficient application.