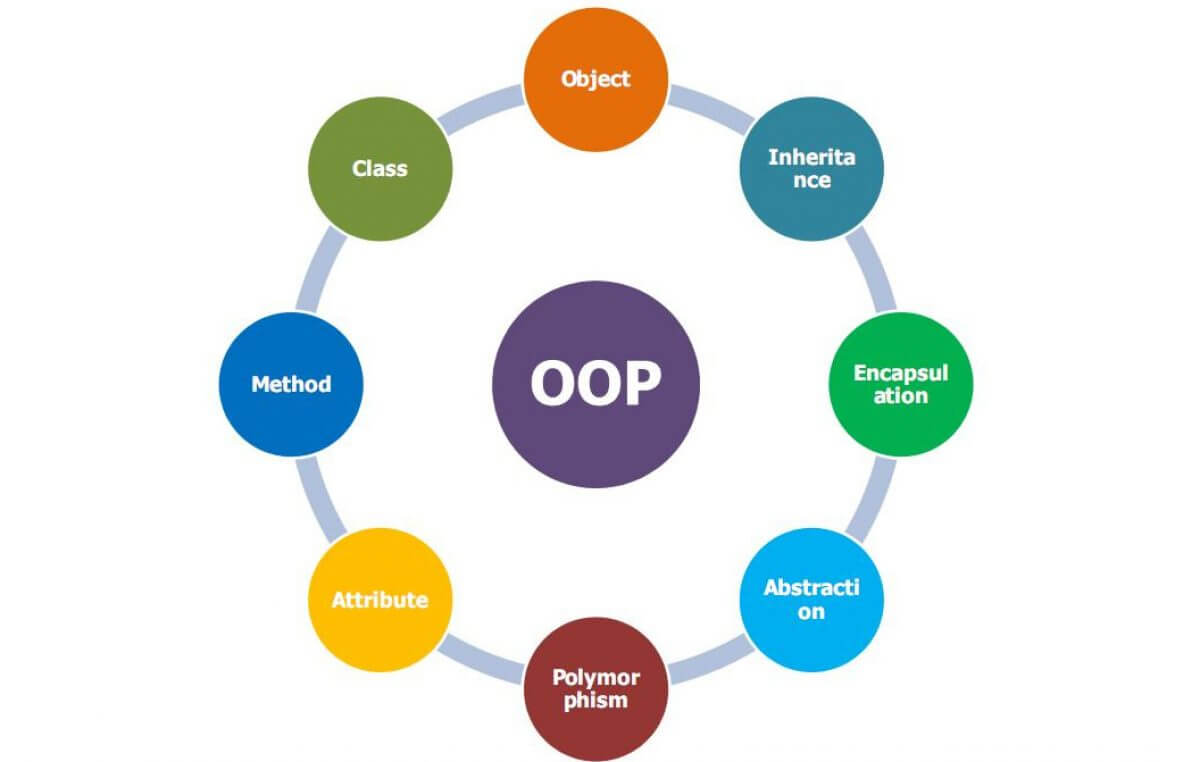
Object-Oriented Programming (OOP) is a programming paradigm that uses objects and classes to organize and structure code. OOP is widely used in many languages, such as Java, C++, Python, and C#. Here are the pros and cons of using OOP:
Pros of Object-Oriented Programming (OOP)
- Modularity and Reusability:
- Encapsulation: OOP allows you to bundle data and functions that operate on that data within a single unit, called a class. This makes it easier to manage and reuse code.
- Code Reusability: Through inheritance, you can create new classes based on existing ones, which reduces code duplication and promotes reusability.
- Maintainability:
- Easier Maintenance: OOP makes it easier to update and maintain code because each object is independent. Changes made to one class don’t affect other parts of the code unless there’s direct interaction. This improves the overall maintainability of the application.
- Modularity: Code is organized into smaller, manageable pieces (objects and classes), making it easier to understand, debug, and extend.
- Scalability:
- OOP promotes scalability by allowing developers to create classes and objects that can easily be extended or modified. As your application grows, new features can be added with minimal changes to existing code.
- Real-World Modeling:
- Modeling Real-World Concepts: OOP allows you to model real-world entities as objects with properties (attributes) and behaviors (methods). This approach makes it easier to conceptualize and design complex systems.
- Abstraction: OOP enables abstraction, which allows you to hide the complex implementation details and expose only essential information to the user. This simplifies the interface and reduces complexity.
- Data Protection and Security:
- Encapsulation: By hiding the internal state of an object and only exposing a public interface, OOP promotes better data security and integrity. This helps in protecting sensitive data from unauthorized access.
- Code Organization:
- OOP naturally encourages better code organization and structuring through the use of classes, objects, and methods. This results in cleaner and more organized code.
- Collaboration:
- OOP makes team development easier. Since objects are modular, multiple developers can work on different parts of the system (different objects or classes) simultaneously without interfering with each other’s work.
- Inheritance and Polymorphism:
- Inheritance allows for code reuse by inheriting properties and behaviors from other classes.
- Polymorphism enables objects of different classes to be treated as objects of a common superclass, making code more flexible and adaptable to changes in requirements.
Cons of Object-Oriented Programming (OOP)
- Complexity:
- Initial Learning Curve: For beginners, OOP can be challenging to understand due to concepts like inheritance, polymorphism, abstraction, and encapsulation. Designing an object-oriented system requires a good understanding of these principles.
- Overhead: For simple programs or small-scale applications, OOP might introduce unnecessary complexity. The need to design classes, define relationships, and manage multiple objects can make small projects more complex than they need to be.
- Performance Overhead:
- Memory Consumption: Objects typically require more memory than simple data structures like arrays. The overhead of storing additional object metadata (e.g., methods and properties) can lead to higher memory usage, especially in large-scale applications with many objects.
- Execution Speed: The use of inheritance, polymorphism, and dynamic dispatch can introduce performance overhead. In some cases, OOP-based systems may be slower than procedural programming approaches, particularly in resource-constrained environments.
- Increased Code Size:
- OOP often requires more lines of code due to the need for additional classes, methods, and objects. This can result in larger codebases, which may be harder to maintain and understand, particularly if the design isn’t well-organized.
- Overuse of Inheritance:
- Tight Coupling: Excessive use of inheritance can lead to tight coupling between classes, making it difficult to modify or extend the system without affecting other parts of the application. If not carefully designed, inheritance hierarchies can become overly complex and hard to manage.
- Difficulty in Understanding Large Systems:
- Complex Interactions: In large systems, understanding how objects interact with each other can be difficult, especially if the system has many interconnected objects or deep inheritance chains. This can lead to a steep learning curve for new developers joining the project.
- Over-Engineering:
- There’s a tendency to over-engineer solutions by trying to model every aspect of the problem with objects. This can result in unnecessary abstractions and complicated designs, which may not be appropriate for the problem at hand.
- Not Always the Best Fit for All Applications:
- OOP is not always the best paradigm for every type of application. For example, certain tasks or problems that involve simple data manipulation might be better suited to a procedural programming approach, where the focus is on actions rather than data and behavior encapsulation.
- Difficulty with Real-Time Systems:
- OOP might not be ideal for applications where performance and real-time processing are critical (such as embedded systems or high-frequency trading). The overhead of object creation and method calls can be detrimental in scenarios where every microsecond counts.
Conclusion:
Object-Oriented Programming offers many advantages, especially in terms of code organization, maintainability, and scalability. However, it can also introduce complexity and performance overhead, particularly when used inappropriately or for simple projects. Understanding when and how to apply OOP principles is key to maximizing its benefits while minimizing its drawbacks.