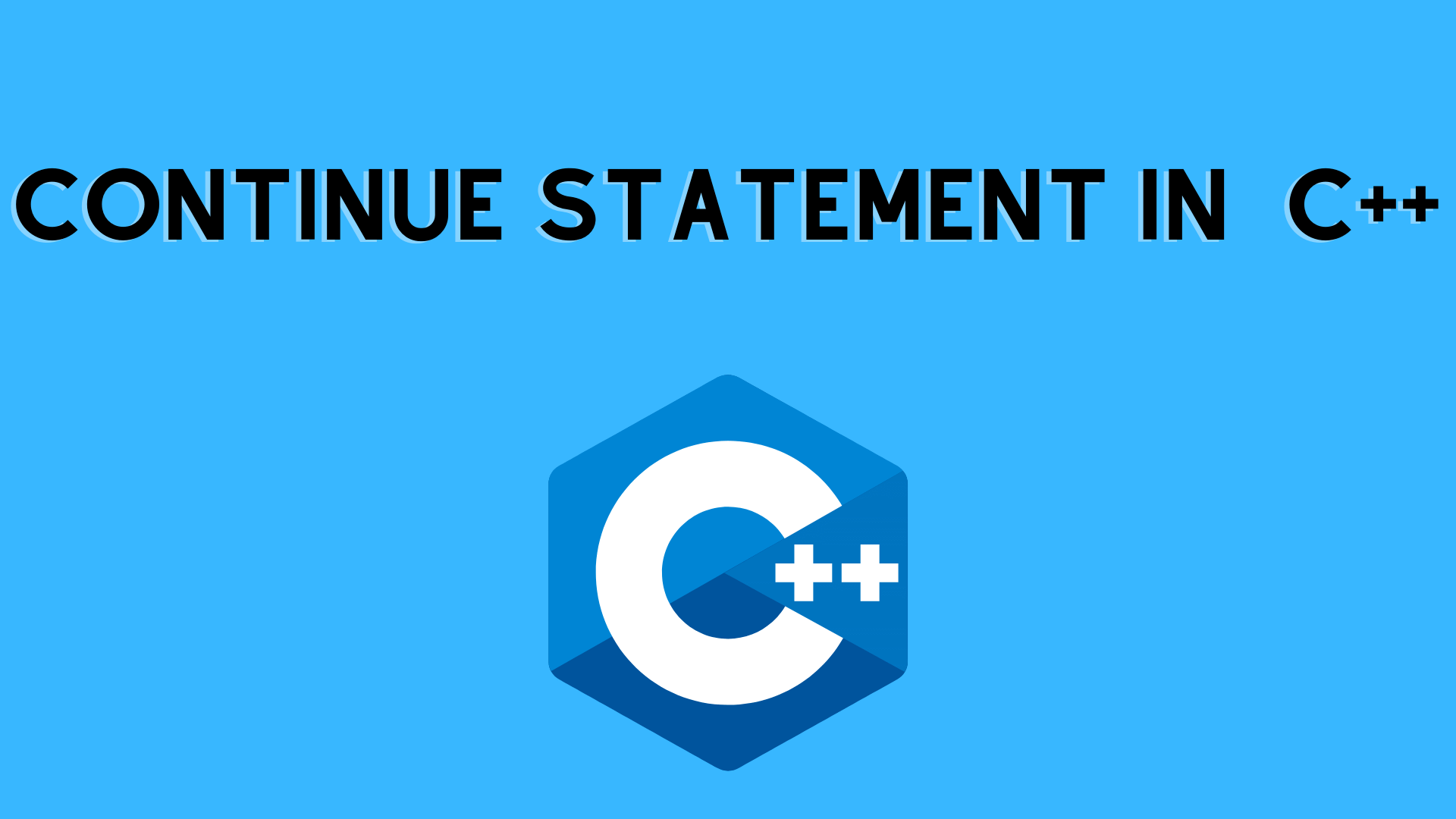
C++ is a powerful and widely-used programming language known for its performance and flexibility. It is particularly well-suited for system programming, game development, and high-performance applications. Here are some of the key strengths of C++:
- High Performance and Efficiency: C++ is a compiled language, meaning that the code is directly translated into machine code, resulting in faster execution compared to interpreted languages. This makes it ideal for performance-critical applications like game engines, real-time systems, and high-frequency trading.
- Object-Oriented Programming (OOP): C++ supports object-oriented programming, which helps in organizing code into reusable objects, classes, and functions. It promotes better code management, modularity, and maintainability, especially in large projects.
- Low-Level Memory Manipulation: C++ gives developers direct control over memory allocation and management via pointers, references, and dynamic memory allocation (using
new
anddelete
). This allows for optimization and fine-grained control over memory usage, which is essential in system-level programming and performance-sensitive applications. - Portability: C++ code can run on multiple platforms (Windows, macOS, Linux, etc.) with minimal changes. The language provides extensive support for cross-platform development, making it ideal for developing applications that need to run on various hardware and operating systems.
- Rich Standard Library: C++ has a rich set of libraries that provide reusable functionality for tasks such as input/output operations, string manipulation, containers (e.g., vectors, maps), algorithms, and more. The Standard Template Library (STL) provides powerful data structures and algorithms for efficient coding.
- Multi-Paradigm Language: While C++ is primarily known for object-oriented programming, it also supports procedural, generic, and functional programming styles. This flexibility allows developers to choose the programming paradigm that best suits the problem at hand.
- High Control Over System Resources: C++ allows direct interaction with hardware and system resources, making it suitable for developing operating systems, device drivers, embedded systems, and other low-level applications. The language provides fine-grained control over system resources like CPU, memory, and I/O.
- Compatibility with C: C++ is backward compatible with C, meaning that C code can often be integrated into C++ programs. This compatibility makes C++ a natural choice for projects that need to combine high-performance, low-level programming with modern software development practices.
- Efficient Use of Resources: Because C++ gives developers control over memory management, it allows for efficient use of resources. This is crucial for applications that need to run on limited hardware or handle large amounts of data, such as embedded systems, gaming, and real-time applications.
- Template Metaprogramming and Generics: C++ supports templates, which allow for the creation of generic functions and classes. Template metaprogramming enables developers to write highly reusable and type-safe code that can work with any data type or class. This reduces the need for code duplication and increases flexibility.
- Concurrency and Multi-Threading: C++ provides support for multi-threading and concurrent programming, allowing developers to create applications that take full advantage of multi-core processors. The language provides various concurrency mechanisms, including thread management and synchronization primitives.
- Error Handling: C++ provides robust exception handling with
try
,catch
, andthrow
statements. This allows developers to manage errors and exceptions effectively, improving the reliability and stability of applications. - Strong Community and Ecosystem: C++ has a large and active developer community, which means that developers can easily find resources, tutorials, libraries, and support. Many well-established tools, frameworks, and engines (such as Unreal Engine, Qt, and Boost) are built using C++, making it easier to develop complex applications.
- Real-Time Systems Support: C++ is frequently used in developing real-time systems that require deterministic performance, such as embedded systems, robotics, and simulation software. Its low-level control, high performance, and efficient memory management make it an excellent choice for such applications.
- Wide Industry Adoption: C++ is used in a wide range of industries, including finance (for high-frequency trading), gaming (for game engines), automotive (for embedded systems and control systems), aerospace (for simulation software), and more. The language’s flexibility and performance have made it a standard in many domains.
- Legacy and Established Codebase: Many critical and large-scale systems, including operating systems, databases, and game engines, have been built with C++ over the years. This has created a vast pool of existing libraries, tools, and expertise that make working with C++ both efficient and practical.
- Large Ecosystem of Tools and Libraries: C++ has a wide array of development tools, compilers, debuggers, and profiling tools that help developers write, debug, and optimize code effectively. Libraries like Boost and frameworks like Qt make it easier to build cross-platform applications and take advantage of C++’s powerful features.
These strengths make C++ an excellent choice for building high-performance applications, system-level software, games, and applications that require direct hardware access or complex computational tasks. Its combination of power, flexibility, and control over system resources makes it one of the most widely used and respected programming languages in the world.